Asserts > Alerts
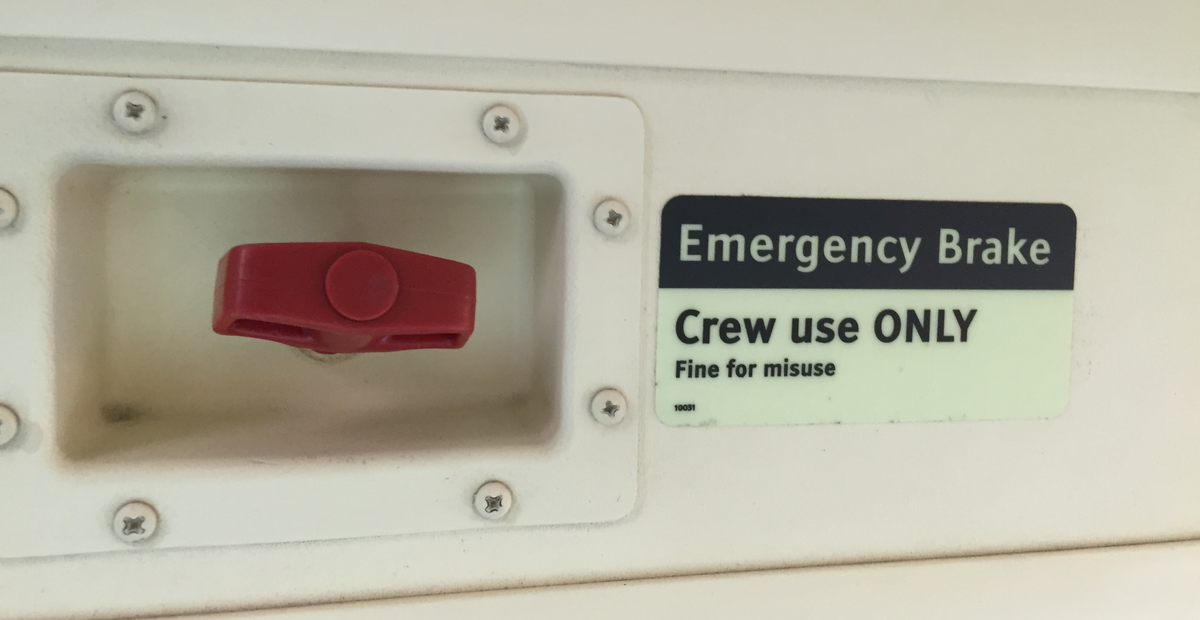
Justin Searls wrote a great piece on how to reduce the risks of writing dangerous code. It’s succinct, focused on a specific problem, and broadly applicable.
His technique relies on assertions, a programming tool that forces failures early when assumptions turn out to be wrong. Assertions don’t just warn you; they stop execution outright, preventing silent errors from spreading.
What if businesses treated critical assumptions the same way?
In code, an assertion crashes a program when something impossible happens. In business, a good assertion forces action before things spiral out of control.
Here’s a highly simplified example:
def always_returns_five():
return 5
x = always_returns_five()
assert x == 5
This function always returns 5, until it doesn’t. If an external API call gets involved, or someone modifies the function, an assertion forces us to stop and investigate immediately, rather than letting a silent failure corrupt the system.
In a code review, assertions can settle debates about assumptions:
- ”What happens if X is false?"
- "That can’t happen."
- "Okay, either add an assertion that crashes the program if you’re wrong, or handle the edge case. Pick one."
Assertions expose when "that can’t happen" really means "I don’t want to write error-handling code."
But this concept doesn’t just apply to software.
Every business process is built on assumptions. And when those assumptions fail, the impact can be massive.
In code, an assertion crashes a program when something impossible happens. In business, a good assertion forces action before things spiral out of control.
Business operations are full of hidden assumptions, just like code:
- "Customers will always renew their contracts."
- "Sales will update CRM records on time."
- "Our marketing campaigns won't burn through budget too quickly."
These are all unstated truths, until they fail. And when they fail, we often find out too late.
So why don’t we assert them, the same way we do in code?
For any of this to work, we need to identify those assumptions. That’s not always easy! But it boils down to asking what needs to be true for the work to be successful.
For each of these critical assumptions, set up an assertion to monitor it. It could be in code, but really doesn’t have to be, as long as checking it is systemized.
Lots of times, we get so close to this idea with alerts. Something goes wrong? No problem, here’s an email about it. The only problem is that you get a lot of emails. And maybe have to do things during the day that (gasp!) take you away from your computer. But maybe you have a team of people receiving the email! Great, but did you set up a protocol for who should handle it (and let people know they’re on it) when one of these come in?
Most businesses already have alerts. But alerts are passive. They assume someone will notice, interpret, and act.
- Alert: "Customer churn is increasing." (Email sent. Will anyone act?)
- Assertion: "If churn rises past 5%, freeze acquisition budgets until leadership intervenes." (A concrete action happens by default.)
With alerts, problems often slip through the cracks. With assertions, failure forces a reaction, like a dead man’s switch. This approach ensures that if no one intervenes, a failsafe activates:
- Finance: If an expense report isn’t reviewed in 7 days, it’s auto-rejected.
- HR: If an exit interview isn’t conducted, an anonymous feedback survey is automatically sent.
- Sales: If a deal isn’t updated in CRM within 10 days, it’s flagged as ‘At Risk’ and removed from forecasting.
Assertions, whether in code or in business, feel risky because they demand action. But the real risk isn’t breaking things when an assumption fails.
The real risk isn’t breaking things, it’s letting failures pile up silently until they explode.